A Tour of Go をやる! – その12 「Basic types」 型抜きってあったな… 【Go言語/A Tour of Go】
2018年9月25日GoA Tour of Go,Goのお勉強,Go言語,型,変数Go,A Tour of Go,Goのお勉強,Go言語,型,変数
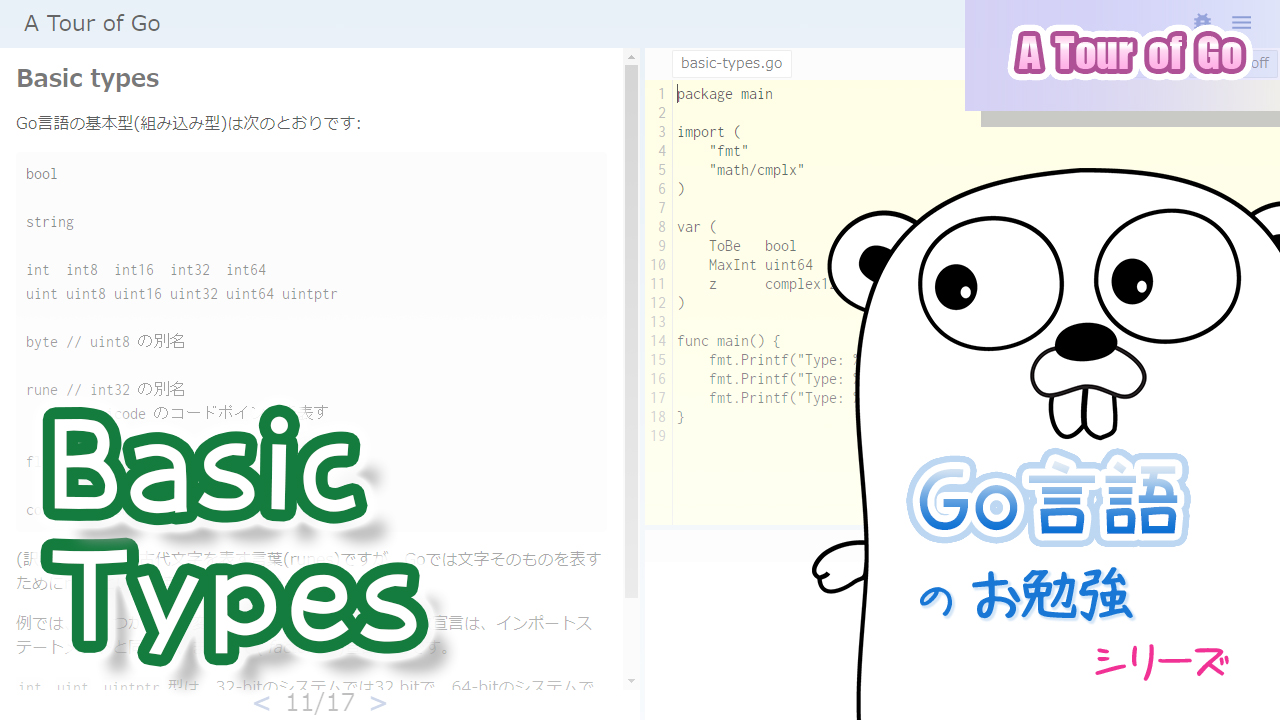
「A Tour of Go をやる!」シリーズの第12回目。
今回も引き続いての変数回、学習内容は型ですぅ~。
今回進めるページ「Basic types」
今回進める「A Tour of Go」のページはこちら。
タイトルの「Basic types」とは?
「Basic types」とありますが、ページの方で紹介されているのはコイツラ。
つまり「Basic types」はコイツラ組み込み型を指しているようです。
bool
string
int int8 int16 int32 int64
uint uint8 uint16 uint32 uint64 uintptr
byte // uint8 の別名
rune // int32 の別名
// Unicode のコードポイントを表す
float32 float64
complex64 complex128
言語仕様の確認
種類が多かったので少し長くなりましたが、各型の仕様は次の通り。
Boolean types
A boolean type represents the set of Boolean truth values denoted by the predeclared constants true and false. The predeclared boolean type is bool; it is a defined type.
ブール型は、事前に宣言された定数trueおよびfalseで示されるブール真理値のセットを表します。事前に宣言されたブール型はboolです。定義された型です。(by Google Translated.)
https://golang.org/ref/spec#Boolean_types
String types
A string type represents the set of string values. A string value is a (possibly empty) sequence of bytes. The number of bytes is called the length of the string and is never negative. Strings are immutable: once created, it is impossible to change the contents of a string. The predeclared string type is string; it is a defined type.
The length of a string s can be discovered using the built-in function len. The length is a compile-time constant if the string is a constant. A string’s bytes can be accessed by integer indices 0 through len(s)-1. It is illegal to take the address of such an element; if s[i] is the i’th byte of a string, &s[i] is invalid.文字列型は、文字列値のセットを表します。文字列値は、(おそらく空の)バイトのシーケンスです。バイト数は文字列の長さと呼ばれ、負になることはありません。文字列は不変です。一度作成すると、文字列の内容を変更することはできません。事前に宣言された文字列型はstringです。定義された型です。
https://golang.org/ref/spec#String_types
文字列の長さsは、組み込み関数lenを使用して検出できます。文字列が定数の場合、長さはコンパイル時定数です。文字列のバイトには、0からlen(s)-1までの整数インデックスでアクセスできます。そのような要素のアドレスを取ることは違法です。 s [i]が文字列のi番目のバイトである場合、&s [i]は無効です。(by Google Translated.)
Numeric types
A numeric type represents sets of integer or floating-point values. The predeclared architecture-independent numeric types are:
数値型は、整数値または浮動小数点値のセットを表します。事前に宣言されたアーキテクチャに依存しない数値タイプは次のとおりです。(by Google Translated.)
https://golang.org/ref/spec#Numeric_types
uint8 the set of all unsigned 8-bit integers (0 to 255)
uint16 the set of all unsigned 16-bit integers (0 to 65535)
uint32 the set of all unsigned 32-bit integers (0 to 4294967295)
uint64 the set of all unsigned 64-bit integers (0 to 18446744073709551615)
int8 the set of all signed 8-bit integers (-128 to 127)
int16 the set of all signed 16-bit integers (-32768 to 32767)
int32 the set of all signed 32-bit integers (-2147483648 to 2147483647)
int64 the set of all signed 64-bit integers (-9223372036854775808 to 9223372036854775807)
float32 the set of all IEEE-754 32-bit floating-point numbers
float64 the set of all IEEE-754 64-bit floating-point numbers
complex64 the set of all complex numbers with float32 real and imaginary parts
complex128 the set of all complex numbers with float64 real and imaginary parts
byte alias for uint8
rune alias for int32
基本となる書き方
コーディングする際の記述例。
// boolean
var [変数名] bool = [値] // bool型 の有効な値は false または true
// e.g.
var tai bool = false
taiyaki := true
// string
var [変数名] string = "[文字列]" // 文字列は""(ダブルクォート)で括る
// e.g.
var ingredients string = "鯛"
cooking := "焼き"
food := ingredients + cooking
// Numeric Types (int, float, etc...)
var [変数名] [数値型] = [数値]
var list_price int = 100
discount_rate := 0.1 // 型は float64
selling_price := float64( list_price ) * ( 1 - discount_rate ) // 型は float64
基本的な型の種類
「A Tour of Go」のページで紹介されていた型の用途をまとめてみます。
型 | 説明 |
---|---|
bool (Boolean) | 真偽値(true / false)を扱う為の型 |
string | 文字列を扱うための型 |
int | 符号付き数値を扱うための型 int8 や int16 の 数字(8 や 16)はビット数のこと、大きいほうが扱える数値の範囲も広い |
uint | 符号なし数値を扱うための型 int と比べると、符号がない分、有効な最大値は大きい |
byte | バイトを扱うための型 byte は uint8 のエイリアス名(1バイト つまり 8bit) |
rune | コードポイントを扱う為の型(コードポイントは Unicode文字 の開始位置) rune は int32 のエイリアス名 |
float | 浮動小数点数を扱うための型 |
complex | 複素数を扱うための型 |
サンプルプログラムを見てみる。
では「A Tour of Go」のサンプルプログラムを見てみます。
今回のお題に関係する箇所にはコメント打ってます。
処理の内容は、幾つかの変数を宣言し初期値を決め、最終的に変数の内容を出力している。
以下はここで使われた型の説明。前の方の項で同様の説明をしたが書いちゃう。
- Boolean型(bool型)
真偽値のことで true あるいは false を値として扱える - uint64型
符号なし数値のことで 0 から 18446744073709551615 までの数値を扱える - complex128型
複素数のことで実数部と虚数部のセットを扱える(実数部や虚数部の有効精度は float64 と同等)
他にも型はありますが、それらの役割や扱える値が知りたければ、言語仕様に詳しく記載されています。
さいごに
今回は Go で扱える型を覚えました。
変数を扱う際にも型指定は必須となるので、しっかり覚えておきたいですな。
私は5分で忘れるけどな…。じゃ、また次回٩( 'ω’ )و
「Go言語のお勉強」インデックス
- うっしー「Go言語」に手を出す…(準備編)
- 「A Tour of Go」をやる!
- その1 「Hello, 世界」
- その2 「Packages」だってさ~
- その3 「Imports」いんぽーっ!
- その4 「Exported names」見えますか?
- その5 「Functions」 これは機能です。
- その6 「Functions continued」(?) 型しょ~略っ!
- その7 「Multiple results」 一粒で二度美味しい?
- その8 「Named return values」 お前は戻り値だ…
- その9 「Variables」入れ物!
- その10 「Variables with initializers」砂でも詰めるか…
- その11 「Short variable declarations」 略パート2!
- その12 「Basic types」 型抜きってあったな…
- その13 「Zero values」 生まれ持った中身は?
- その14 「Type conversions」 1は1.0になれる!
- その15 「Type inference」 君の型は…?
- その16 「Constants」 変えられねぇよ?
- その17 「Numeric Constants」 型無し野郎?
- その18 「For」 ~いよいよループ!~
- その19 「For continued」 ~ Forの続き! ~
「A Tour of Go」を題材にした記事は現在書き直し中…。_(:3」∠)_
ほとんどサボりが原因で尻切れとなっていたシリーズですが、最近になってようやく続きを書きたくなり再開をしようと思ったんです。が、古い記事を見返すとデータ・フォーマットが古かったり内容が分かり難かったりに気づきまして、、、この際だからと最初の記事から書き直しをすることになりました…
関連記事
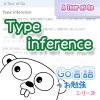
A Tour of Go をやる! – その15 「Type inference」 君の型は…?【Go言語/A Tour of Go】
「A Tour of Go をやる!」シリーズの第15回目。 今回も変数でまたし ...
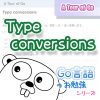
A Tour of Go をやる! – その14 「Type conversions」 1は1.0になれる! 【Go言語/A Tour of Go】
「A Tour of Go をやる!」シリーズの第14回目。 今回も変数…という ...
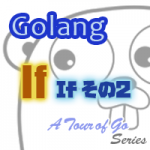
【Go言語】スコープ…エイm? な If - その2
え~、Go です。 前回の続きです。 というわけで、If の使い方その2ってこと ...
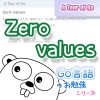
A Tour of Go をやる! – その13 「Zero values」 生まれ持った中身は? 【Go言語/A Tour of Go】
「A Tour of Go をやる!」シリーズの第13回目。 まだまだ続くぞ変数 ...
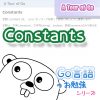
A Tour of Go をやる! – その16 「Constants」 変えられねぇよ? 【Go言語/A Tour of Go】
「A Tour of Go をやる!」シリーズの第16回目。 呆れるほど変数のお ...
ディスカッション
コメント一覧
まだ、コメントがありません